1. Introduction to File Handling
Definition: File handling refers to the process of creating, reading, writing, and managing files stored on your system using Python’s built-in functions.
Importance of File Handling:
- Data Storage: Ensures data persistence beyond program execution.
- Data Exchange: Facilitates sharing information between systems or programs.
- Automation: Speeds up repetitive tasks like logging or report generation.
2. The Basics of Python File Handling
Python makes file handling straightforward and efficient using the built-in open() function. This function is the gateway to interacting with files—reading data, writing to them, or creating new files. Let's explore the open() function first.
2.1 The open() Function
The open() function is used to open a file and return a file object, which serves as a connection to the file. This file object allows you to perform operations like reading, writing, or appending data.
Syntax
file = open("filename", "mode")
Modes of Operation:
Mode | Description |
---|---|
"r" | Read (default mode). Opens a file for reading. |
"w" | Write. Creates a new file or overwrites an existing file. |
"a" | Append. Adds data to the end of the file without overwriting. |
"rb" | Read binary. Opens a file in binary read mode. |
"wb" | Write binary. Opens a file in binary write mode. |
2.2 Reading Files
Using read() Method: Reads the entire content of a file as a string.
with open("example.txt", "r") as file:
content = file.read()
print(content)
Using readline() Method: Reads a single line at a time.
with open("example.txt", "r") as file:
line = file.readline()
print(line)
Using readlines() Method: Reads all lines and returns them as a list.
with open("example.txt", "r") as file:
lines = file.readlines()
print(lines)
2.3. Writing Files
Using write() Method: Writes a string to a file. Overwrites content if the file exists.
with open("output.txt", "w") as file:
file.write("Hello, Python!")
Using writelines() Method: Writes multiple lines to a file.
lines = ["Line 1\n", "Line 2\n", "Line 3\n"]
with open("output.txt", "w") as file:
file.writelines(lines)
2.4 Appending to Files
The "a" mode lets you add content to an existing file without overwriting it.
with open("output.txt", "a") as file:
file.write("\nAppending this line.")
3. Binary File Handling
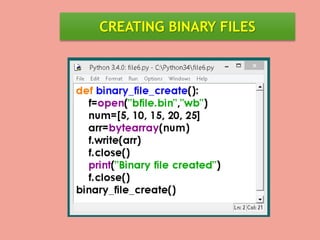
Binary files store data in bytes, useful for non-text files like images or videos.
Opening Binary Files:
file = open("filename", "mode")
Reading Binary Files:
with open("image.jpg", "rb") as file:
content = file.read()
print(content[:10]) # Print first 10 bytes
Writing Binary Files:
with open("copy.jpg", "wb") as file:
file.write(content)
4. Managing File Resources
Python provides a with statement to manage file resources automatically.
Benefits:
- Ensures files are closed after operations.
- Prevents memory leaks or data corruption.
Example:
with open("example.txt", "r") as file:
content = file.read()
# File is closed automatically here
5. File Pointer Operations
Python keeps a file pointer to track where reading/writing occurs.
Seek: Move the pointer to a specific location in the file.
with open("example.txt", "r") as file:
file.seek(5) # Move to the 6th character
print(file.read())
Tell: Get the current position of the file pointer.
with open("example.txt", "r") as file:
print(file.tell()) # Output: Current pointer position
6. File Existence and Removal
Check if a File Exists: Use the os module to verify file existence.
import os
if os.path.exists("example.txt"):
print("File exists!")
else:
print("File not found!")
Deleting a File:
os.remove("example.txt")
7. Error Handling in File Operations
Handle exceptions to ensure smooth execution.
try:
with open("nonexistent.txt", "r") as file:
content = file.read()
except FileNotFoundError:
print("File not found!")
except IOError:
print("Error reading or writing the file.")
Key takeaways:
- Modes: r, w, a, rb, wb determine how a file is accessed.
- File Methods: Use read, write, seek, and tell for efficient operations.
- Best Practices: Always use with for safer file handling and manage exceptions effectively.
Next Topic : Important Python Libraries