1. Introduction to Sets
A set is a built-in data structure in Python that stores unique and unordered items. Inspired by mathematical sets, Python sets offer powerful operations for managing collections of distinct elements.
1.1 What is a Set?
A set is an unordered collection where:
- Items are unique (duplicates are automatically removed).
- Elements can be of any hashable type, such as integers, strings, or tuples.
- Sets are mutable, meaning you can add or remove items, but the elements themselves must be immutable.
1.2 Syntax
Sets are defined using curly braces {} or the set() function.
# Creating sets
my_set = {1, 2, 3} # Using curly braces
empty_set = set() # Using the set() constructor
invalid_set = {} # Creates an empty dictionary, not a set
mixed_set = {1, "Python", (2, 3)} # Sets can store elements of different types
2. Key Characteristics of Sets
Unordered
- Elements in a set do not have a defined order.
- Indexing and slicing are not supported.
my_set = {1, 2, 3} print(my_set[0]) # Error! Sets do not support indexing
Unique Elements
- Duplicate elements are removed automatically.
my_set = {1, 2, 2, 3} print(my_set) # Output: {1, 2, 3}
- Mutable
- Sets can be modified by adding or removing elements.
- Hashable Elements Only
- Elements of a set must be immutable, such as numbers, strings, and tuples. Lists or dictionaries cannot be part of a set.
3. Creating Sets
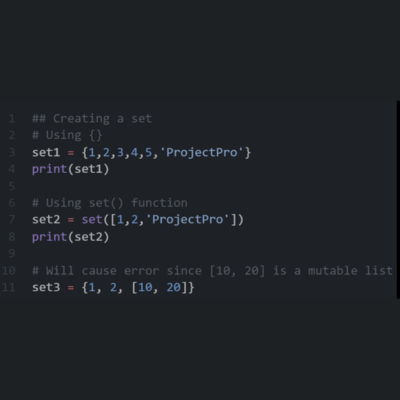
3.1 Using Curly Braces {}
This is the most common way to create a set.
my_set = {1, 2, 3}
print(my_set) # Output: {1, 2, 3}
3.2 Using the set() Constructor
Useful when creating a set from an existing iterable like a list or string.
# From a list
list_data = [1, 2, 2, 3]
my_set = set(list_data)
print(my_set) # Output: {1, 2, 3}
# From a string
string_data = "hello"
my_set = set(string_data)
print(my_set) # Output: {'h', 'e', 'l', 'o'}
3.3 Empty Set
To create an empty set, use the set() constructor.
empty_set = set()
print(empty_set) # Output: set()
4. Adding and Removing Elements
4.1 Adding Elements
- add(item): Adds a single element to the set.
my_set = {1, 2}
my_set.add(3)
print(my_set) # Output: {1, 2, 3}
4.2 Removing Elements
- remove(item): Removes the specified element. Throws an error if the element is not found.
my_set = {1, 2, 3}
my_set.remove(2)
print(my_set) # Output: {1, 3}
- discard(item): Removes the specified element, but does not throw an error if it doesn’t exist.
my_set.discard(4) # No error, even though 4 is not in the set
- pop(): Removes and returns an arbitrary element from the set.
my_set = {1, 2, 3}
popped_item = my_set.pop()
print(popped_item) # Output: 1 (or another element; it's arbitrary)
- clear(): Removes all elements, leaving the set empty.
my_set = {1, 2, 3}
my_set.clear()
print(my_set) # Output: set()
5. Mathematical Set Operations
Python sets support a variety of operations based on mathematical set theory.
5.1 Union
Combines all unique elements from two sets. Use | or the union() method.
set1 = {1, 2, 3}
set2 = {3, 4, 5}
print(set1 | set2) # Output: {1, 2, 3, 4, 5}
print(set1.union(set2)) # Output: {1, 2, 3, 4, 5}
5.2 Intersection
Finds common elements between two sets. Use & or the intersection() method.
set1 = {1, 2, 3}
set2 = {3, 4, 5}
print(set1 & set2) # Output: {3}
print(set1.intersection(set2)) # Output: {3}
5.3 Difference
Finds elements in the first set but not in the second. Use - or the difference() method.
set1 = {1, 2, 3}
set2 = {3, 4, 5}
print(set1 - set2) # Output: {1, 2}
print(set1.difference(set2)) # Output: {1, 2}
5.4 Symmetric Difference
Finds elements in either set but not in both. Use ^ or the symmetric_difference() method.
set1 = {1, 2, 3}
set2 = {3, 4, 5}
print(set1 ^ set2) # Output: {1, 2, 4, 5}
print(set1.symmetric_difference(set2)) # Output: {1, 2, 4, 5}
6. Useful Set Methods
6.1 Membership Test
Check if an element exists in a set using in or not in.
my_set = {1, 2, 3}
print(2 in my_set) # Output: True
print(4 not in my_set) # Output: True
6.2 Copying a Set
Create a duplicate of a set using the copy() method.
original = {1, 2, 3}
duplicate = original.copy()
print(duplicate) # Output: {1, 2, 3}
7. Applications of Sets
Removing Duplicates:
data = [1, 2, 2, 3, 4] unique_data = set(data) print(unique_data) # Output: {1, 2, 3, 4}
- Mathematical Operations:
Use sets to perform union, intersection, or difference operations on datasets. - Fast Membership Testing:
Sets are optimized for checking if an element exists, making them ideal for such tasks. - Data Cleaning:
Eliminate duplicate or redundant information from a dataset.
8. Comparison with Lists and Tuples
Feature | Set | List | Tuple |
---|---|---|---|
Mutability | Mutable | Mutable | Immutable |
Order | Unordered | Ordered | Ordered |
Duplicates | Not Allowed | Allowed | Allowed |
Use Case | Unique items, fast ops | Sequential data storage | Immutable collections |
Key Takeaways:
- What is a Set?
- Unordered, unique, and mutable collection of elements.
- Creating Sets
- Use {} or set(). For an empty set, use set().
- Characteristics
- Unordered: No indexing/slicing.
- Unique: Removes duplicates automatically.
- Immutable Elements: Only hashable types allowed (e.g., numbers, strings, tuples).
- Manipulating Sets
- Add: add().
- Remove: remove(), discard(), pop(), or clear().
- Operations
- Union: Combine elements (|).
- Intersection: Common elements (&).
- Difference: Unique to the first set (-).
- Symmetric Difference: In either but not both (^).
- Applications
- Removing duplicates, data cleaning, fast membership testing, and performing mathematical operations.
Next Topic : Python Data Structures